之前我们说过,好将CSS样式和JS脚本排入队列,而不是将它们直接硬编码到模板文件中。这是因为编入队列可以提供更大的灵活性。
如果正确完成,编入队列的方式还会告诉 WordPress 正在加载哪些资源。当 WordPress 知道需要哪些资源时,它可以确保相同的资源不会被多次加载。当您有一个非常流行的库(例如 jQuery 或 FontAwesome)且多个主题和插件将使用时,这一点尤其重要。
编入队列的另一个好处是,编入队列的资源可以通过插件被禁用,从而无需修改模板文件。
虽然我们的主题有一个style.css文件,但它还没有使用它,现在让我们将其加入队列,在functions.php添加如下代码,那么在style.css添加的样式将会作用于主题的样式:
function my_custom_theme_enqueue() {wp_enqueue_style( 'my-custom-theme', get_stylesheet_uri() );
}
add_action( 'wp_enqueue_scripts', 'my_custom_theme_enqueue' );
get_stylesheet_uri()
是一个辅助函数,用于检索当前主题样式表的 URI。如果我们要对任何其他文件进行排队,我们需要这样做:
wp_enqueue_style( 'my-stylesheet', get_template_directory_uri() . '/css/style.css' );
我们的主题没有任何脚本,如果有,我们将像这样将它们排队:
function my_custom_theme_enqueue() {
wp_enqueue_style( 'my-custom-theme', get_stylesheet_uri() );
wp_enqueue_script( 'my-scripts', get_template_directory_uri() . '/js/scripts.js' );
}
add_action( 'wp_enqueue_scripts', 'my_custom_theme_enqueue' );
上述情况的一个例外是 WordPress预先注册的脚本,在这种情况下,您只需提供第一个参数 ($handle):
wp_enqueue_script( 'jquery' );
在functions.php文件中把所有的路劲都写好:(以下代码来源于WP大学https://www.wpdaxue.com/docs/theme-handbook/basics/including-css-javascript)
function add_theme_scripts() {
wp_enqueue_style( 'style', get_stylesheet_uri() );
wp_enqueue_style( 'slider', get_template_directory_uri() . '/css/slider.css', array(), '1.1', 'all');
wp_enqueue_script( 'script', get_template_directory_uri() . '/js/script.js', array ( 'jquery' ), 1.1, true);
if ( is_singular() && comments_open() && get_option( 'thread_comments' ) ) { wp_enqueue_script( 'comment-reply' );}
}
add_action( 'wp_enqueue_scripts', 'add_theme_scripts' );

然后在header.php通过<?php wp_head(); ?>
引用就可以。(<?php wp_head(); ?>
除了引用样式,还引用标题等其他元素,安装的很多插件都依赖这个hook)
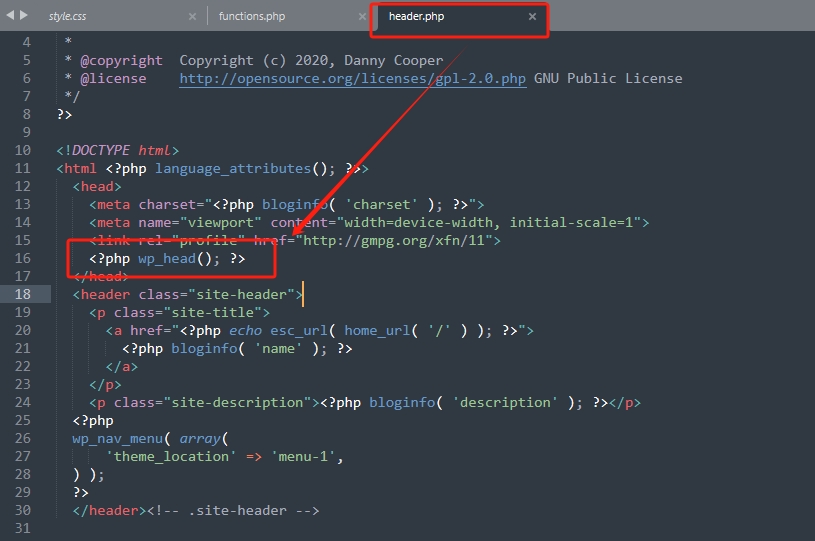
代码详细注释版本:
<?php
// 函数用于添加CSS样式表到WordPress队列
function enqueue_custom_styles() {
// 参数依次为样式的标识符(自定义名称)、样式表的路径、依赖关系(如果有)、版本号,是否在页脚加载
wp_enqueue_style('custom-style', get_stylesheet_directory_uri() . '/css/custom-style.css', array(), '1.0.0', 'all');
}
// 将enqueue_custom_styles函数挂载到wp_enqueue_scripts动作上,确保在WordPress加载样式表时调用
add_action('wp_enqueue_scripts', 'enqueue_custom_styles');
// 函数用于添加JS脚本到WordPress队列
function enqueue_custom_scripts() {
// 参数依次为脚本的标识符(自定义名称)、脚本文件的路径、依赖关系(如果有)、版本号,是否在页脚加载
wp_enqueue_script('custom-script', get_template_directory_uri() . '/js/custom-script.js', array('jquery'), '1.0.0', true);
}
// 将enqueue_custom_scripts函数挂载到wp_enqueue_scripts动作上,确保在WordPress加载脚本时调用
add_action('wp_enqueue_scripts', 'enqueue_custom_scripts');
?>
上述代码中的注释解释了每个函数的用途和参数,以下是对注释的进一步解释:
enqueue_custom_styles
函数:wp_enqueue_style
: WordPress函数,用于添加CSS样式表到队列。'custom-style'
: 样式表的标识符,可用于在后续的调用中引用该样式表。get_stylesheet_directory_uri() . '/css/custom-style.css'
: 样式表的路径,这里使用了主题的样式表目录。array()
: 依赖关系,如果有其他样式表依赖于此样式表,可以在这里列出。'1.0.0'
: 样式表的版本号,有助于浏览器缓存刷新。'all'
: 样式表的媒体类型,可以是 ‘all’, ‘screen’, ‘print’ 等。
enqueue_custom_scripts
函数:wp_enqueue_script
: WordPress函数,用于添加JS脚本到队列。'custom-script'
: 脚本的标识符,可用于在后续的调用中引用该脚本。get_template_directory_uri() . '/js/custom-script.js'
: 脚本文件的路径,这里使用了主题的脚本目录。array('jquery')
: 依赖关系,如果脚本依赖于其他脚本(例如 jQuery),可以在这里列出。'1.0.0'
: 脚本的版本号,有助于浏览器缓存刷新。true
: 表示将脚本放置在页面底部,以确保在页面内容加载完成后再加载脚本。
enqueue_custom_styles
和enqueue_custom_scripts
函数可以合并在一起写,这样可以更清晰地组织代码。以下是合并后的代码:
<?php
// 函数用于添加CSS样式表和JS脚本到WordPress队列
function enqueue_custom_styles_and_scripts() {
// 添加CSS样式表到队列
wp_enqueue_style('custom-style', get_stylesheet_directory_uri() . '/css/custom-style.css', array(), '1.0.0', 'all');
// 添加JS脚本到队列
wp_enqueue_script('custom-script', get_template_directory_uri() . '/js/custom-script.js', array('jquery'), '1.0.0', true);
}
// 将enqueue_custom_styles_and_scripts函数挂载到wp_enqueue_scripts动作上,确保在WordPress加载样式表和脚本时调用
add_action('wp_enqueue_scripts', 'enqueue_custom_styles_and_scripts');
?>
这样的合并代码将两个函数的内容整合到一个函数中,使代码更加简洁而且易于维护。同时,只需一个add_action
调用即可确保在WordPress加载样式表和脚本时调用该函数。